Typically, LinqConnect generates a separate Table property for each entity type in the custom DataContext class. An exception from this rule is entity types that form an inheritance hierarchy. In this case, LinqConnect uses single Devart.Data.Linq.Table for all types in the hierarchy (regardless the inheritance is Table-Per-Hierarchy or Table-Per-Type). In particular, persisting new entities should be done via this Table of the base type.
For example, let us pick out all discontinued products as a subtype of the Product entity type:
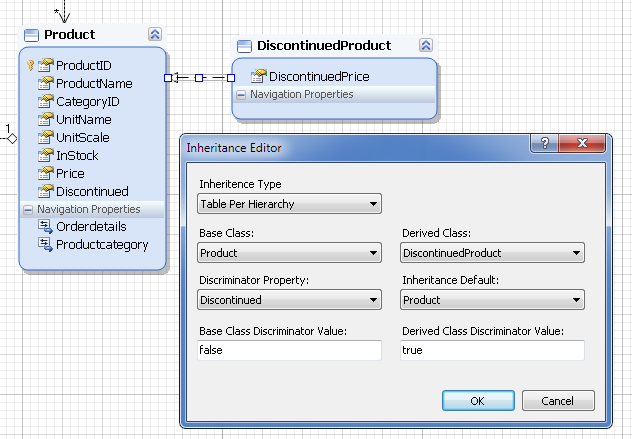
If we insert a new product object, we do this via the Table of the Product type, whether or not the new entity is DiscontinuedProduct:
C#csharp | ![]() |
---|---|
Product newProduct = new Product() { ProductID = 9010, ProductName = "R. Temam. Infinite Dimensonal Dynamical Systems.", CategoryID = 9, Price = 90, InStock = 50 }; DiscontinuedProduct newDiscontinued = new DiscontinuedProduct() { ProductID = 9011, ProductName = "R. Temam, Navier-Stokes Equations.", CategoryID = 9, Price = 80, DiscontinuedPrice = 60, InStock = 12 }; context.Products.InsertOnSubmit(newProduct); context.Products.InsertOnSubmit(newDiscontinued); context.SubmitChanges(); |
Visual Basic | ![]() |
---|---|
Dim newProduct As New Product() With { _ .ProductID = 9010, _ .ProductName = "R. Temam. Infinite Dimensonal Dynamical Systems.", _ .CategoryID = 9, _ .Price = 90, _ .InStock = 50 _ } Dim newDiscontinued As New DiscontinuedProduct() With { _ .ProductID = 9011, _ .ProductName = "R. Temam, Navier-Stokes Equations.", _ .CategoryID = 9, _ .Price = 80, _ .DiscontinuedPrice = 60, _ .InStock = 12 _ } context.Products.InsertOnSubmit(newProduct) context.Products.InsertOnSubmit(newDiscontinued) context.SubmitChanges() |
![]() |
---|
On an attempt to use the Table of the inherited type, InvalidOperationException is thrown. (It is actually not obvious how one can even try using the 'inherited' table - the corresponding property isn't even generated, right? - but recall that the 'Products' property is a wrapper on the GetTable<Product>() method, thus one can try executing the GetTable<DiscontinuedProduct>() method.) The exception text will be "Could not retrieve a Table for inheritance subtype 'CrmDemoContext.DiscontinuedProduct', try Table of CrmDemoContext.Product instead." |